Write Windows Plugins using DLL
Write a plugin using Dynamic-link library (DLL) and monitor data based on your need. You can also write plugins using Batch, PowerShell, and VB scripts.
Prerequisites
- Latest version of our Windows server monitoring agent
- Plugin must be written in C# (.NET framework 3.0 and above)
Write a Plugin
- Download and install the latest version of the Windows agent in your server
- Open Visual Studio Editor > Create a Project > Select Language Visual C# > Class Library for creating C# class library > OK
- Add reference to the created project. Go to Projects menu > Add Reference. Select Site24x7Plugin.Monitor.dll from the agent installation folder C:\Program files (x86)\Site24x7\WinAgent\monitoring\Plugins\PluginsReference.
Note
Site24x7Plugin.Monitor.dll' contains interface IPlugin which contains the mandatory variables and methods which should be implemented in creates project's c# class file.
- In the project's C# class file, add namespace using Site24x7Plugin.Monitor and implement the class using IPlugin Interface.
- Mandatory Variables to be present in a class:
- Version: Any change in the plugin script file would need an update to the plugin version. The default value is 1. After any change to an existing plugin, the user must increment the version number by 1. Version should be whole numbers only. E.g. 1,2,3
- Implement the method DataCollect() and write your logic to get the actual data. This method should return Dictionary<string, object> which should contain key value pairs. The key should the name of the metric.
- Optional Variables to be present in a class:
- Heartbeat: Alerts the plugin as down, when no data is received from the plugin by the Site24x7 data center. Value for heartbeat should be either true or false. By default, the value of heartbeat is true
- DisplayName: Name of the monitor as displayed in the web client. For eg: if you create a plugin called "Site24x7Plugin", your plugin class should have a display name whose return value must be "Site24x7Plugin"
- msg: Assign the error messages that are captured when there is any error thrown during DataCollect() method to this variable
- The properties Version, Displayname and Heartbeat will return a string object
- Optional Methods to be present in a class:
- The Units() method, should return a Directory<string, object> which should contain a key value pair. The keys should have the name of the attributes to be monitored and the values should be their respective units.
- Build the project by going to Visual Studio > Build menu > Build Solution. A .dll file would be created.
- Create a folder under Agent installation directory/Monitoring/Pluginsand copy the .dll file in this folder.
Note
Make sure the name of the file and the folder name, both are identical. For eg., If you are writing a plugin for Apache, the folder name should be Apache and the plugin script file should be named as apache.dll.
- Our agent will start collecting data and within five minutes, the user will be able to see the stats in our webclient. What could be the next possible steps after successfully adding a plugin?
All plugin logs will be captured in files PluginLog.log and PluginRegisterLog.log under [installation directory]\monitoring\logs\Details
Sample Plugin File
using System;
using System.Collections.Generic;
using System.Text;
using Site24x7Plugin.Monitor;namespace Plugin
{
public class test : IPlugins
{
public string DisplayName
{
get{ return "File system performance"; }
}
public string Version
{
get { return "1"; }
}
public string Heartbeat
{
get { return "True"; }
}
public object Units()
{
IDictionary<String, object> sample = new Dictionary<String, object>();
sample.Add("File Control Bytes Per Second", "Bytes/sec");
sample.Add("File Control Operations Per Second", "operations/sec");
sample.Add("File Data Operations Per Second", "Bytes/sec");
sample.Add("File Read Bytes Per Second", "Bytes/sec");
return sample;
}
public object DataCollect()
{
Random r = new Random();
IDictionary<String, object> sample = new Dictionary<String, object>();
sample.Add("File Control Bytes Per Second", 10);
sample.Add("File Control Operations Per Second", 4);
sample.Add("File Data Operations Per Second", 1.2);
sample.Add("File Read Bytes Per Second", 87);
return sample;
}
}
}
JSON output:
{
"data":{"File Control Operations Per Second":4,"File Data Operations Per Second":1.2,"File Read Bytes Per Second":87,"File Control Bytes Per Second":10},
"units":{"File Control Operations Per Second":"operations/sec","File Data Operations Per Second":"Bytes/sec","File Read Bytes Per Second":"Bytes/sec","File Control Bytes Per Second":"Bytes/sec"},
"type":"Plugin.dll",
"version":"1",
"availability":"1"
}
Data as represented in the Site24x7 web client:
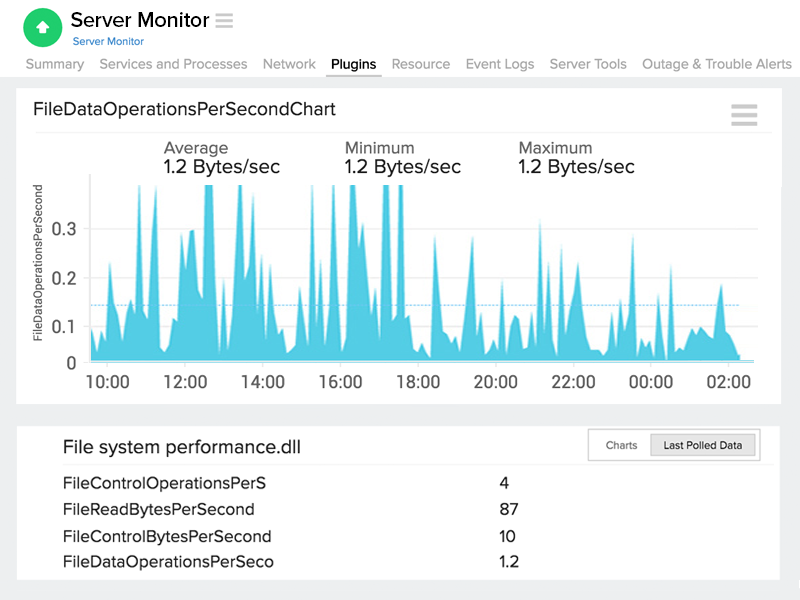
Troubleshooting Tips
- How many plugins can I add per server monitor?
- Possible reasons why my Windows plugin is not added to my account
- How to debug my plugin monitor?
- Possible steps to be performed if your plugin is in the suspended state
- What happens if I delete a plugin monitor?