iOS APM
Site24x7 Mobile APM for iOS lets you track the performance of your native mobile applications on actual end-user devices. For example, a news reader application may perform the following operations internally:
- Navigate to a table view to show a list of articles
- Load the list of articles using a REST API call
- Cache the list of articles in an SQLite database
- Download a thumbnail for each article
- Cache the thumbnails to the filesystem
- Build a complex UI such as a custom table view cell style
All of the above are potentially long-running operations that impact user experience so it's important to benchmark and optimize them across various devices. Site24x7 Mobile APM gathers and aggregates metrics from all your users across the globe by embedding an APM agent in your applications in the form of a library.
The APM agent measures the execution time of your code using transactions and components. In the previous example, the entire sequence of operations from starting navigation to rendering the final UI can be considered a transaction. The individual operations can be grouped into different component types such as HTTP, SQLite, filesystem, UI etc. Simple operations can be measured using just transactions, while complex operations can be measured using transactions with components.
Learn about:
Add Mobile APM?
- Login to Site24x7 and go to iOS section under APM tab.
- Click on Add Application button.
- Enter the Application Name and Apdex Threshold and click Save.
- You can copy the application key by clicking on the hamburger icon (
) near your app name in the application screen.
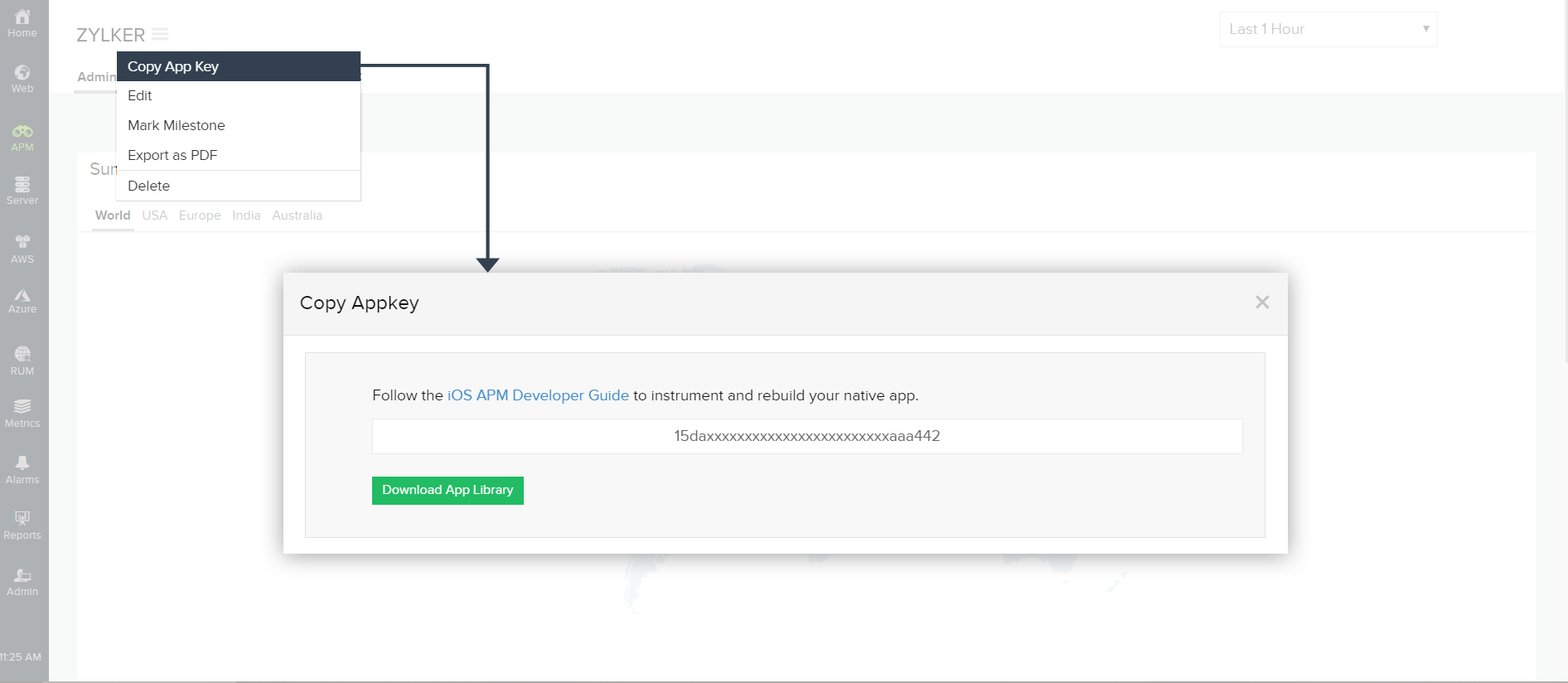
Install Mobile APM via cocopods
Step 1:
Add Mobile APM iOS SDK to your podfile.
target 'Your_Project_Name' do
use_frameworks!
pod 'Site24x7APM'
end
Step 2:
Run the below command in your terminal
pod install
Step 3:
Set Debug Information format as DWARF with dysm File in Build Settings.
Step 4:
Initialise your SDK and build your project .
import UIKit
import APM
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
S24APM.start(withAppKey:"App_Key", interval: 15);
return true;
}
}
Start a transaction
You should typically start a transaction before a long-running operation and stop it when the operation is complete. Transactions are thread-safe and can be started and stopped from different threads. A transaction object can only be started and stopped once. Transactions with the same name are averaged across the application. Thus, when the same operation is executed multiple times using the same transaction name, the average execution time is recorded.
Group operations within a transaction using components?
You can group operations within a transaction into different types of components. You can use one of the predefined types such as S24HttpComponent.TYPE_HTTP, Component.TYPE_SQLITE, Component.TYPE_UI etc., or specify your own type. Components are thread-safe and can be started and stopped from different threads. A component object can only be started and stopped once. A component object cannot be stopped after its parent transaction has already been stopped. Multiple components in a transaction can overlap and run in parallel. Components with the same name within a transaction are averaged.
In the above example, the total time taken by HTTP operations (downloading articles and thumbnails) are measured by S24HttpComponent and the time taken for just the articles is measured by "Download Articles". The time taken for each thumbnail is averaged and recorded by "Download Thumbnail" since it executes multiple times within a loop.
Flush the data?
Sometimes it's desirable to manually flush recently recorded data to Site24x7's servers. You may want to do this if you record transactions just before your application is terminated. If you've set a large upload interval (the default is 60 seconds), you should manually flush data whenever appropriate in case the application is terminated before the next upload interval.
[S24APM flush]
View the data
- Log in to your Site24x7 account > APM > iOS and click on your application.
- The dashboard lists all your mobile apps along with their statuses: Up, Down, Critical, or Trouble.
- Applications that have been suspended or have configuration errors are also listed here.
- For active applications, the following metrics are listed in the dashboard:
- Response time and throughput for the chosen time period compared with the previous time period
- Crashes for the chosen time period
- Apdex score and user count for the chosen time period
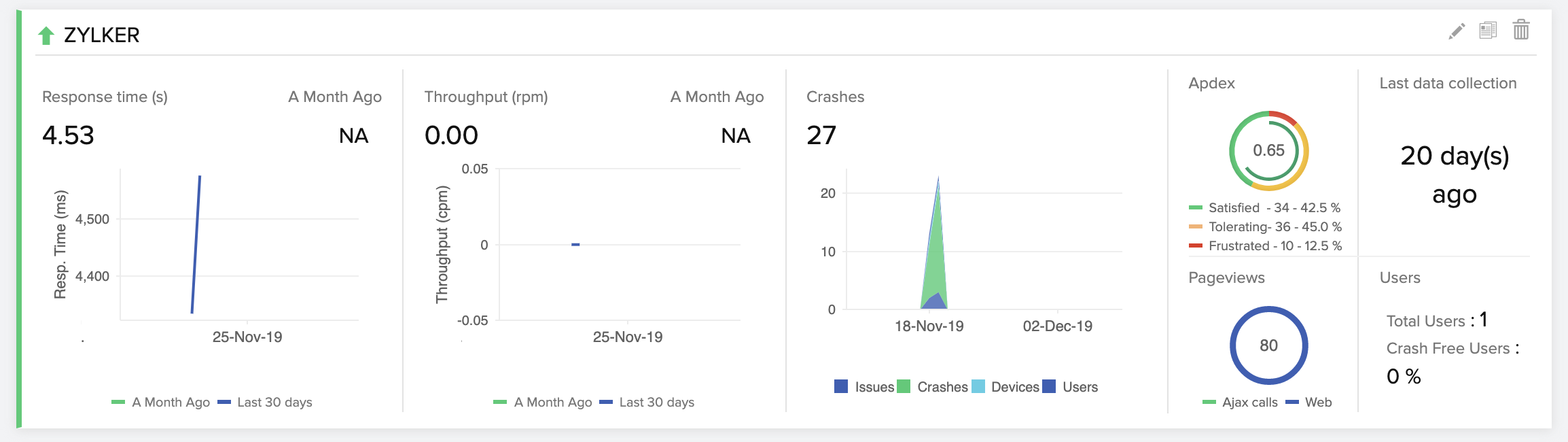
Metrics and Inference
- Worldwide summary
- Response time and throughput
- Response time by geographies, carriers, devices, and OSs
- Total response time, throughput, and response time split up of individual transactions
- Crash analytics
1.Worldwide summary report
The worldwide summary report shows the performance of your application across all geographies for the chosen time period. The color code is based on the Apdex score, ranging from 0 to 1, with 0 denoting a frustrating user experience and 1 denoting a satisfied user experience. You can hover over an individual region on the map to view the Apdex score, average response time, throughput, and errors for that particular location.
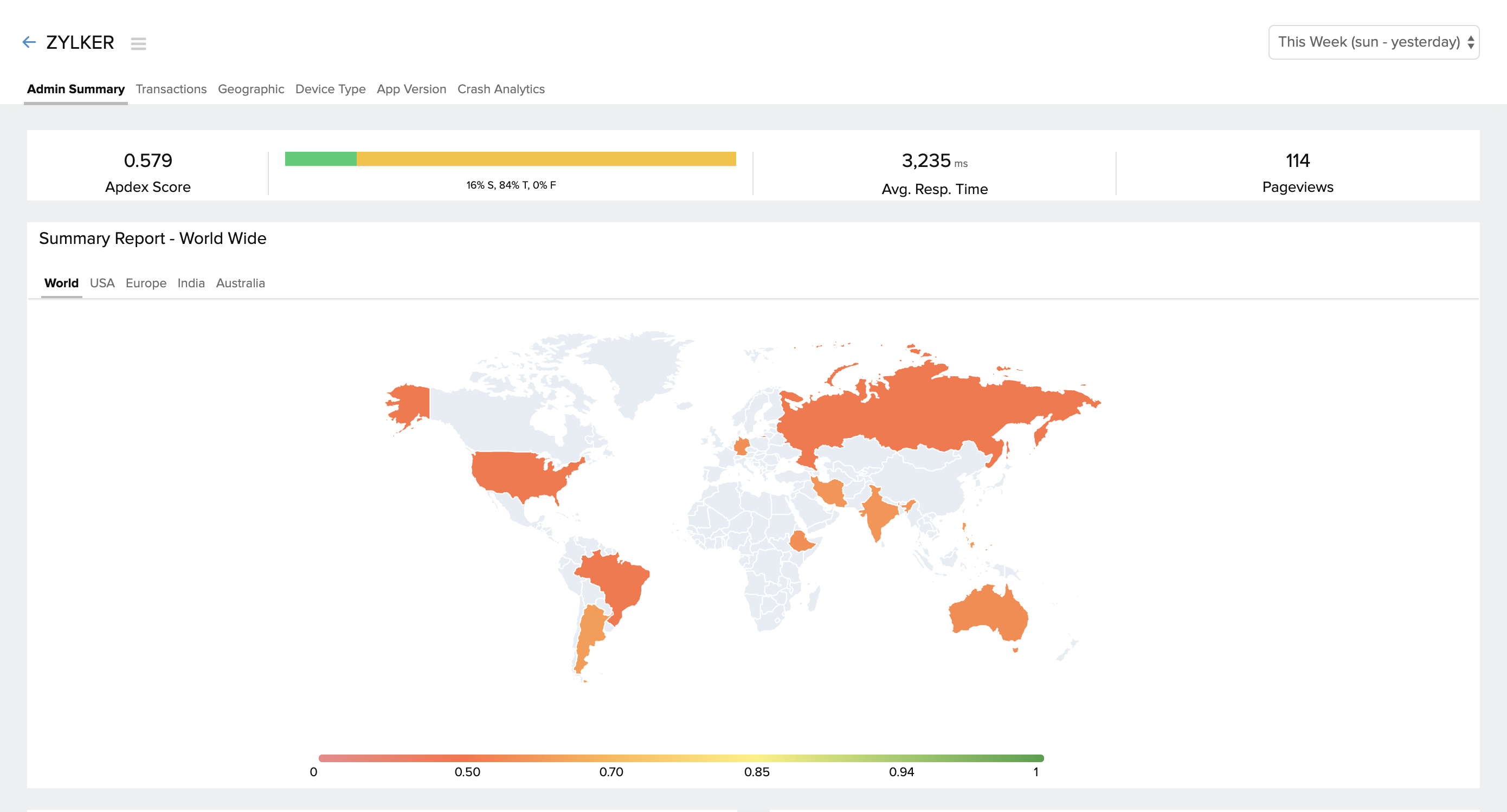
2.Response time and throughput of the app
The total response time and throughput of the app for the chosen time period can be viewed from the Admin tab.
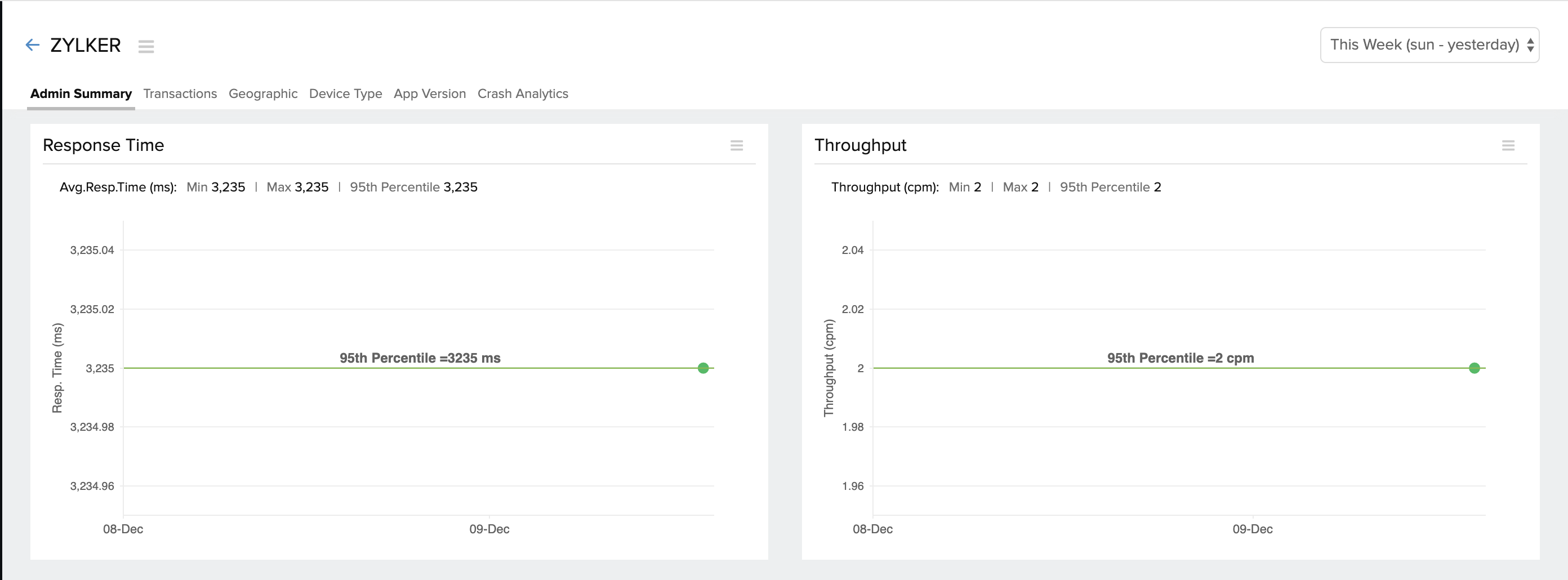
3.Response time split up by geography, carrier, device, and OS version
Your application response time may be impacted by various reasons other than your application code.
Comparing your app response time across geographies, devices, and OS versions will help you narrow down the issue.
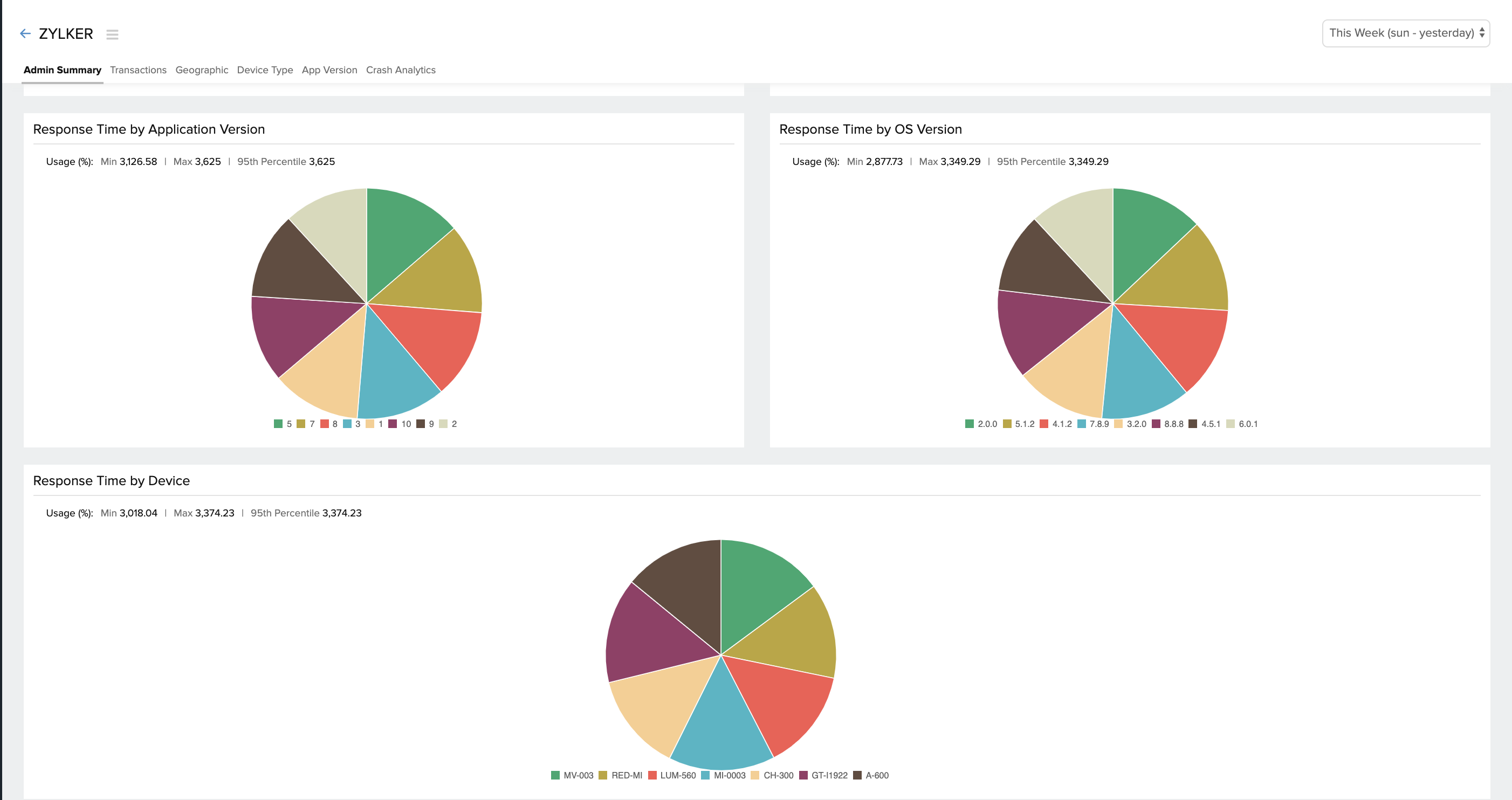
Response time by geographies and carriers can be viewed under the Geography tab.
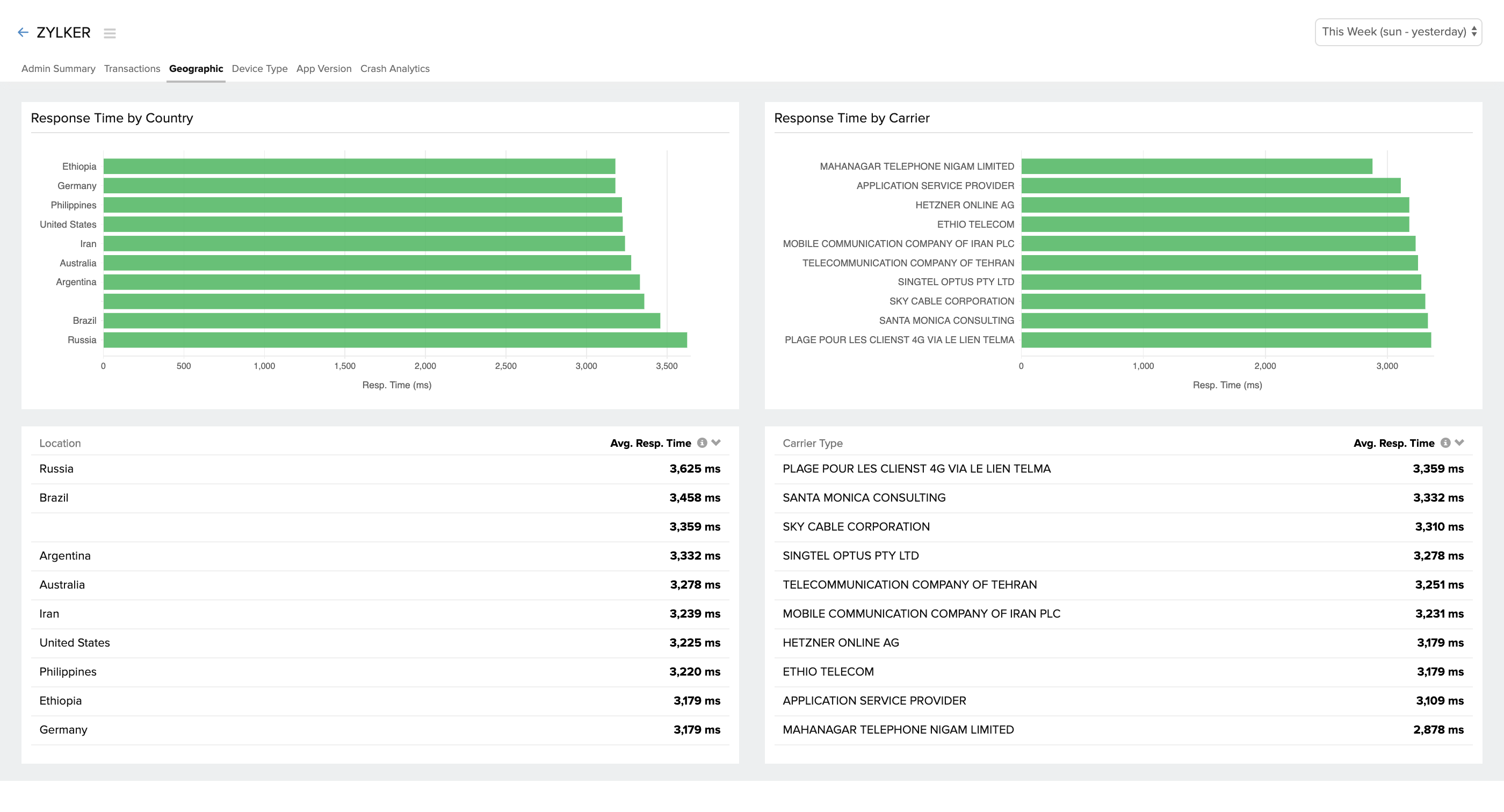
Response time across various devices and OS versions can be seen under the Device Type tab.
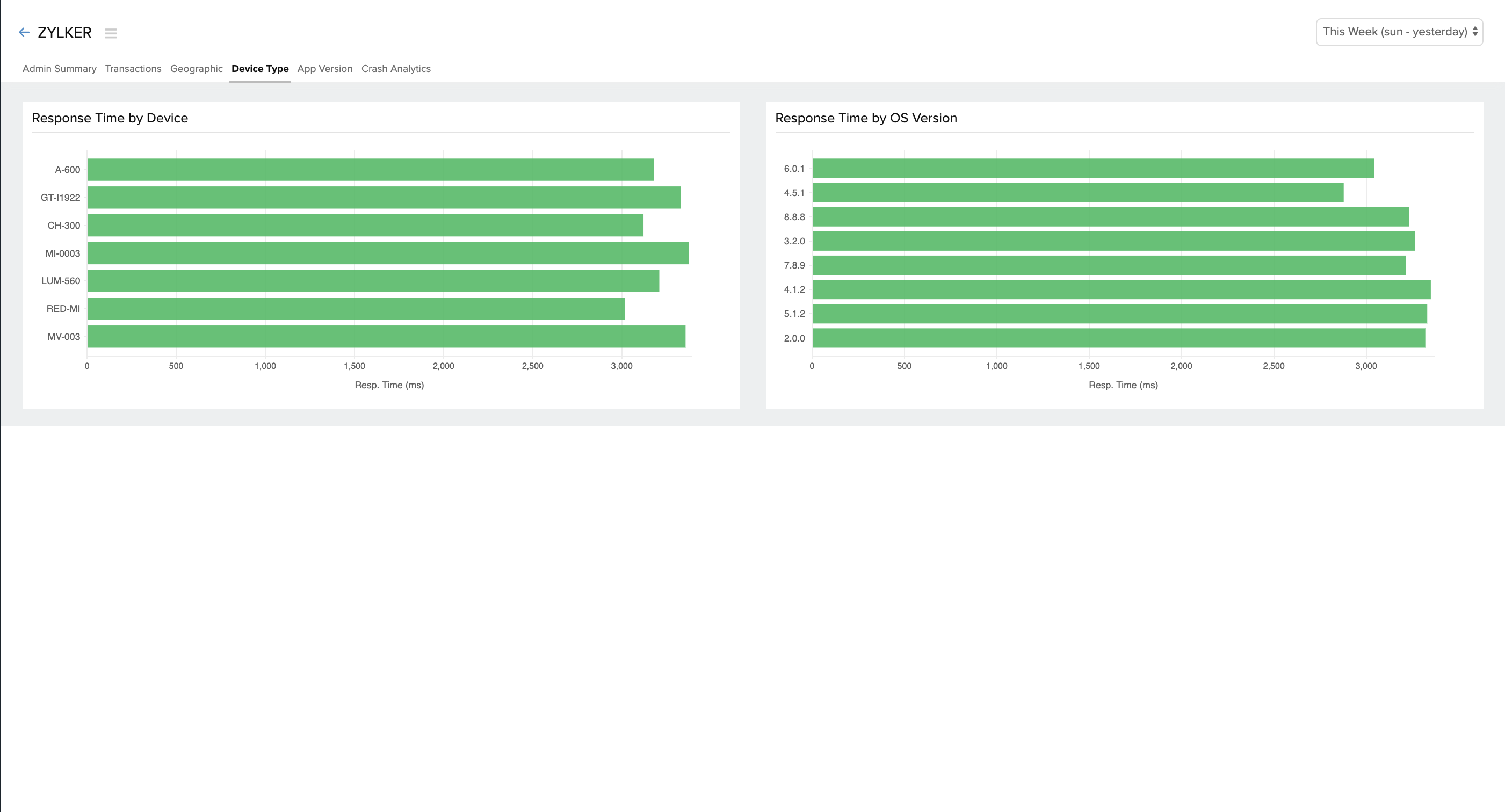
4.Response time of transactions
Response time, throughput, and count (the number of times that transaction was called) of individual transactions can be viewed under the Transactions tab.
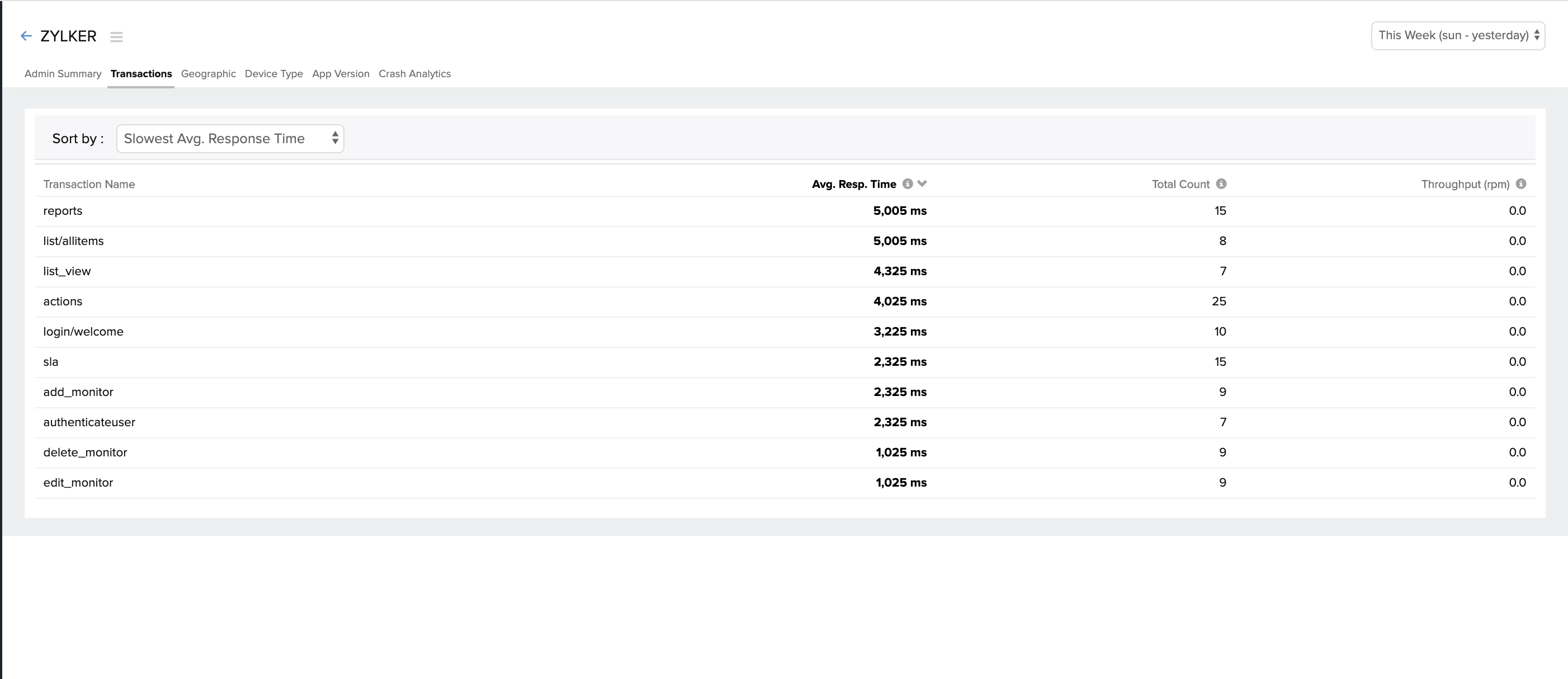
5.Crash Analytics
You can view the number of crashes for the chosen time period by clicking on the Crash Analytics tab. You can hover over the stacked graph on the top, to view the number of crashes across various devices, the number of issues, and the number of users who were affected by this crash. The middle band shows you the percentage change in the crashes — an increase or decrease in the total number of crashes along with the count of crash-free users.
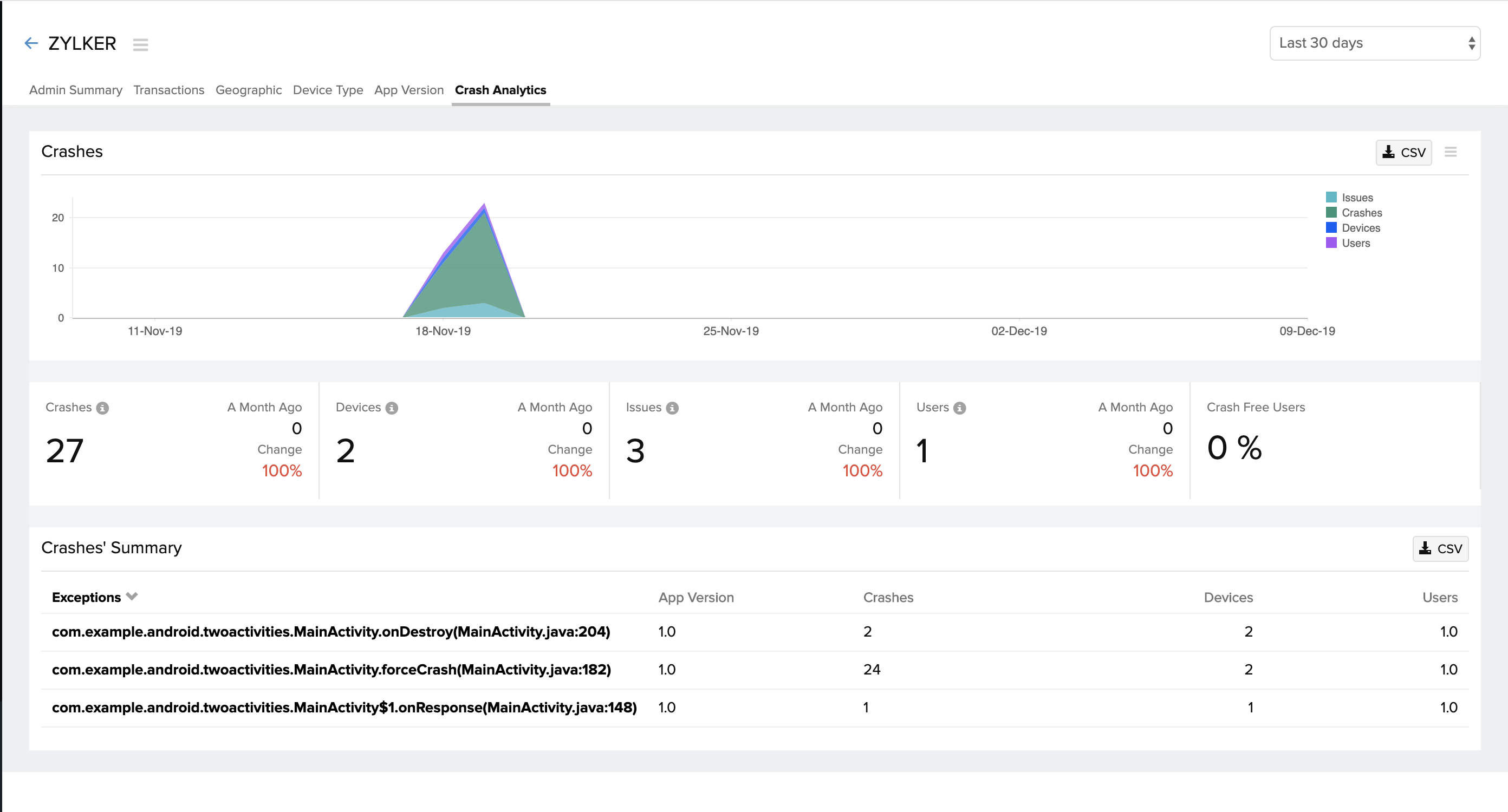
The crash summary lists the exceptions. You can click on the exception to find the exact line of error.
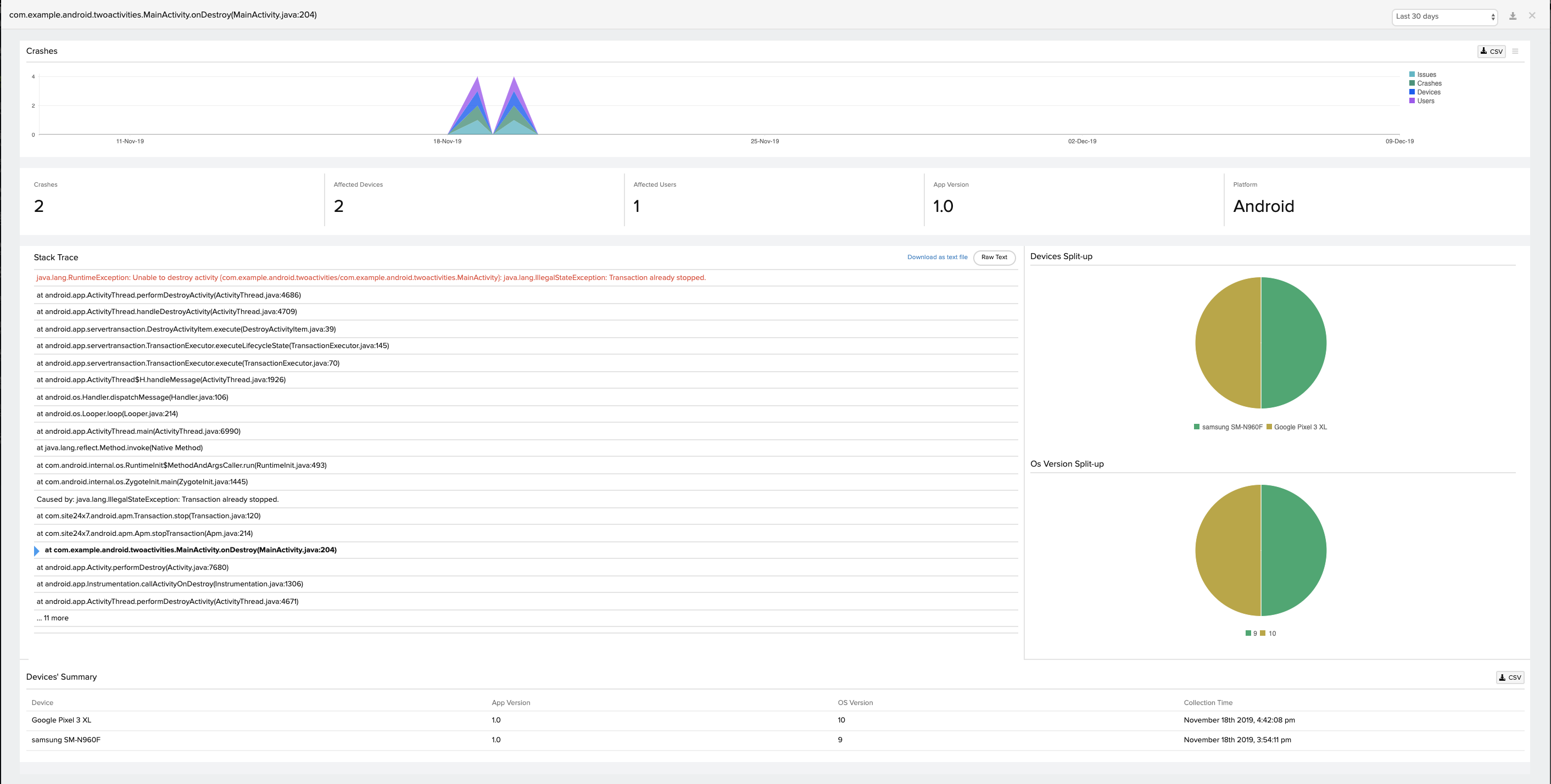
Configure Alerts
Get notified when your application response time exceeds the specified threshold level, by configuring alerts.
You can set alerts as Trouble or Critical based on the severity level.
Follow the steps below to configure alerts:
- Log in to your Site24x7 account > APM > iOS.
- Select your application > Hover over the hamburger icon > Edit monitor details.
- Under Configuration Profiles, click on (+) to add a new threshold and availability profile.
- Select your mobile application in Monitor Type and give a name for your alert.
- Enter the average response time value - you will receive alerts when your application response time exceeds this value.
- Click Save
You can tweak the settings in Notification profile, to decide when to receive alerts when your application goes DOWN. Additionally, you can also configure user alerts groups - this lets you decide who should receive alerts when triggered.
Custom APIs
Custom APIs are used to set dynamic values for user IDs, track custom events, capture screens, and more. This document will explain the various types of custom APIs available in Site24x7 and the syntax to use them.
Available APIs:
- Initializing the SDK
- Custom User ID
- Transactions
- HTTP Call
- Screen
- Environment
- Flush
- Exclude HTTP Calls
- Exclude Screens
1. Initializing the SDK
You can use the API below to initialize the SDK with a custom upload interval. By default, the data upload interval is set to 60 seconds.
Syntax for Objective-C:
Objective-C
/**
* Initializes the APM agent.
* @param {NSString*} appKey
* The application key.
* @param {NSUInteger} intervel
* The interval in seconds at which to upload data to the server. The default is 60 seconds.
*/
[S24APM startWithAppKey:@"App_Key" interval:30]
Syntax for Swift:
Swift
/**
* Initializes the APM agent.
* @param {String} appKey
* The application key.
* @param {int} intervel
* The interval in seconds at which to upload data to the server. The default is 60 seconds.
*/
S24APM.start(withAppKey:"App_Key", interval: 15);
2. Custom User ID
By default, a unique user ID is generated by the SDK. If you want to customize user IDs, you can do so by implementing the following syntax. This comes in handy when you want to track metrics or debug issues specific to a particular user.
Syntax for Objective-C:
Objective-C/**
*@param {NSString*} userId
*/
[S24APM setUserId:@"user@example.com" ]
Syntax for Swift:
Swift
/**
*@param {String} userId
*/
S24APM.setUserId("user@zylker.com")
3. Transactions
You can use the API below to track custom events with application-specific timing.
Syntax for Objective-C:
Objective-C
/**
*Transaction (can contain multiple components)
*@param {NSString*} transactionName
*Component
*@param {NSString*} transactionName
*@param {NSString*} componentName
*/
S24Transaction *transaction = [S24APM startTransactionWithName:@"List Articles"];
S24Component *httpComponent = [transaction startComponentWithType:S24HttpComponent];
S24Component *articlesComponent = [transaction startComponentWithType:@"Download Articles"];
// Download articles
[transaction stopComponent:articlesComponent];
for (Article *article in articles) {
S24Component *thumbnailComponent = [transaction startComponentWithType:@"Download Thumbnail"];
// Download thumbnail
[transaction stopComponent:thumbnailComponent];
}
[transaction stopComponent:httpComponent];
[S24APM stopTransaction:transaction];
Syntax for Swift:
Swift
/**
*Transaction (can contain multiple components)
*@param {String} transactionName
*Component
*@param {String} transactionName
*@param {String} componentName
*/
S24Transaction *transaction = [S24APM startTransactionWithName:@"List Articles"];
S24Component *httpComponent = [transaction startComponentWithType:S24HttpComponent];
S24Component *articlesComponent = [transaction startComponentWithType:@"Download Articles"];
// Download articles
[transaction stopComponent:articlesComponent];
for (Article *article in articles) {
S24Component *thumbnailComponent = [transaction startComponentWithType:@"Download Thumbnail"];
// Download thumbnail
[transaction stopComponent:thumbnailComponent];
}
[transaction stopComponent:httpComponent];
[S24APM stopTransaction:transaction];
4. HTTP Call
By default, network requests that use the following networking libraries are automatically captured.
- NSUrlSession
- NSUrlConnection
If you want to add more HTTP calls, use the API below.
Syntax for Objective-C:
Objective-C
/**
*@param {NSString*} name
*@param {NSUInteger} duration measured in millis
*@param {NSString*} startTime
*@param {NSUInteger} respCode
*@paramt {NSString*} httpMethod
*/
[S24APM addHttpCall:@"example.com" withTime:60 startTime:@"1642750130310" respCode:200 httpMethod:@"GET"]
Syntax for Swift:
Swift
/**
*@param {String} name
*@param {int} duration measured in millis
*@param {String} startTime
*@param {int} respCode
*@paramt {String} httpMethod
*/
S24APM.addHttpCall("example.com", withTime:60, startTime: "1642750130310", respCode: 200, httpMethod: "GET");
5. Screen
By default, all screens are captured; however, if you want to add a missing screen, use the API given below.
Syntax for Objective-C:
Objective-C
/**
*@param {NSString*} screen
*@param {NSUInteger} duration measured in millis
*@param {NSString*} startTime
*/
[S24APM addScreen:@"DetailScreen" withTime:60 startTime:@"1642750130310"]
Syntax for Swift:
Swift
/**
*@param {String} screen
*@param {int} duration measured in millis
*@param {String} startTime
*/
S24APM.addScreen("DetailScreen", withTime: 60, startTime: "1642750130310");
6. Environment
You can use the API below to set custom environment details for filtering data across various environment setups like development, debug, production, or release.
Syntax for Objective-C:
Objective-C
/**
*@param {NSString*} environment
Custom environment types like debug, release, or beta release.
*/
[S24APM setEnvironment:@"release"]
Syntax for Swift:
Swift
/**
*@param {String} environment
Custom environment types like debug, release, beta-release, etc.
*/
S24APM.setEnvironment("release");
7. Flush
You can use the API below to immediately upload recorded data to the server instead of waiting for the next upload interval. By default, the flush interval is set to 60 seconds.
Syntax for Objective-C:
Objective-C
[S24APM flush]
Syntax for Swift:
Swift
S24APM.flush()
If there's no network available, data will be held in the queue until the next upload interval. If you've configured a long upload interval, you may need to manually flush data when your activities or services are destroyed.
8. Exclude HTTP Calls
You can exclude custom URLs from tracking by passing a list of URLs as an argument to the API below.
Syntax for Objective-C:
Objective-C
/**
@param {NSArray<NSString *> *} listOfUrls
*/
[S24APM enableHttpTracking:@[@"example.com"]]
Syntax for Swift:
Swift
/**
@param {var} listOfUrls
*/
S24APM.enableHttpTracking(["example.com"]);
9. Exclude Screens
You can exclude custom screens from tracking by passing a list of screens as an argument to the API below.
Syntax for Objective-C:
Objective-C
/**
@param {NSArray<NSString *> *} listOfScreens
*/
[S24APM ignoreScreens:@["DetailScreen"]]
Syntax for Swift:
Swift
/**
@param {var} listOfScreens
*/
S24APM.ignoreScreens(["DetailScreen"]);
Release Notes
Version 2.2.3
10 June 2024
Issue fix:
- Fixed the issue with the ignoreScreens custom API.
Version 2.2.2
03 June 2024
Enhancement:
- Privacy Manifests are included as part of Apple's security regulations.
Version 2.2.1
05 February 2024
Enhancement:
- Support for the Flutter framework.
Version 2.2.0
21 December 2023
New Features:
The following are the mobile vitals features:
- Support for capturing application start up time (both warm and cold launches).
- Support for automatically calculating slow & frozen frames for view controllers.
Enhancements:
- The session time has been limited to 15 minutes.
- The application version is taken from CFBundlShortVersionString.
- Support for the CocoaPods platform.
Version 2.1.2
05 June 2023
Enhancements:
- Some of the logic is moved to Swift using bridge headers.
- A null safety check is performed, before sending data to servers.
- The Singleton Pattern is used throughout the SDK to share objects.
Issue Fix:
- The issue of being unable to write error data to file has been resolved.
Version 2.1.1
17 May 2023
Issue Fixes:
- Crash data sent to native iOS agent from React Native applications is saved to a file just before the application crashes.
- When the application starts, a task will be launched to send the previously stored error data to our servers.
- After the agent successfully communicates with the server, the error file from the application storage will be cleared.
Version 2.1.0
20 May 2022
New Feature:
- New API for monitoring the application using the app key and upload interval.
Enhancement:
- Enhanced Crashlytics support.
Version 2.0.4
04 March 2022
Enhancement:
- Support for HTTP calls in Swift-based iOS applications.
Version 2.0.3
19 January 2022
Issue Fixes:
- Minor bug fixes.
Version 2.0.2
12 January 2022
Enhancements:
- For each upload interval time, the most latest 20 screens and HTTP calls are captured.
- Introduced XCFrameworks, which supports both simulator and real devices.
- API for configuring a custom environment.
Version 2.0.1
11 January 2022
Issue Fix:
- The "UI API was called on a background thread" issue has been resolved.
Version 2.0.0
04 June 2021
Enhancements:
- Support for tracking HTTP calls with the request method, response time, throughput, status codes, platform, and screens.
- Support for tracking screens based on the response time, throughput, and count.
- Support for automatic tracking of views and navigations.
- Support for manually tracking views.
- Tracking of user sessions is enabled, with a session timeout of 60 minutes.
- Support for manually adding user IDs.
- Support for tracking all HTTP calls for the following packages:
- NSURLSession methods
- dataTaskWithURL
- dataTaskWithURL completionHandler
- dataTaskWithRequest
- dataTaskWithRequest completionHandler
- downloadTaskWithURL
- downloadTaskWithURL completionHandler
- downloadTaskWithRequest
- downloadTaskWithRequest completionHandler
- uploadTaskWithRequest fromData
- uploadTaskWithRequest fromData completionHandler
- uploadTaskWithRequest fromFile
- uploadTaskWithRequest fromFile completionHandler
- NSURLSession methods
-
- NSURLConnection methods
- sendAsynchronousRequest queue completionHandler
- dataTaskWithURL completionHandler
- NSURLConnection methods
- Support for manually adding any other HTTP calls.